Number Guessing Game Mini Project using C with Source Code
Contents
Introduction
The C programming language is a procedural programming language. Between 1969 and 1973, Dennis Ritchie worked on the project. It was created primarily as a system programming language for developing operating systems. Low-level memory access, a small collection of keywords, and a clean style are all qualities that make C language excellent for system programming, such as operating system or compiler development.
Why Learn C Programming Language?
Because it mixes the characteristics of high-level languages with the functionalism of assembly language, C is often referred to as a middle-level computer language. The manipulation of bits, bytes, and addresses in C gives the programmer more control over how the program will behave and more direct access to the underlying hardware mechanisms.
Working programmers influenced, influenced, and field-tested C. As a result, C provides the programmer with exactly what he or she want. C++ is a more advanced version of the C programming language. C++ incorporates all of the features of C, as well as support for object-oriented programming (OOP). Furthermore, C++ includes numerous enhancements and features that make it a “better C,” regardless of whether or not it is used for object-oriented programming.
Number Guessing Game
The best way to learn is to do. Hence, what better way to learn C programming language (if you know the basics) than to code your first mini project. For this blog we are going to build number guessing game mini project using c programming language. Keep in mind this is a mini project for absolute beginners and intermediate programmers as well.
Explanation
The concept for our guessing game can be understood using the below example
An example of how our game works:
Player A: I am thinking of a number from 1 to 100, can you guess my number within 4 turns ?
Player B: Sure, is your number 50 ?
Player A: Nope too high !!.
Player B: Okay, is your number 25 ?
Player A: Nope, guess a higher number.
Player B: Okay is your number 37 ?
Player A: Nope, guess a higher number.
Player B: Okay, is your number 45 ?
Player A: Yes, congratulations you guessed my number in 4 turns you win!
Our Approach
- The user specifies the range’s lower and upper bounds.
- The compiler creates a random integer in the range and saves it in a variable for future use.
- A while loop will be set up to allow for repeated guessing.
- If the user guesses a number that is higher than a randomly chosen number, the user is given the warning “You guessed too high !!”
- Else If the user guesses a number that is less than the randomly chosen number, the user receives the message “You guessed too low !!”
- And if the user guessed in a minimum number of guesses, the user gets a congratulatory “You guessed the correct number !!!!!!!” output along with the number of guesses.
Source Code
#include<stdio.h>
#include<stdlib.h>
#include<time.h>
int main()
{
int number, guess, no_of_guesses=1;
srand(time(0));
// Generates a random number between 1 and 100 or even more if you wish
number = rand()%100 + 1;
// Keep running the loop
// until the number is guessed
do
{
printf("Guess the number between 1 to 100 !!!!!!\n");
scanf("%d", &guess);
if(guess>number)
{
printf("You guessed to high !!\n");
}
else if(guess<number)
{
printf("You guessed too low !!\n");
}
else
{
printf("You guessed the correct number !!!!!!! \n");
printf("Number of attempts : %d, Not bad !!", no_of_guesses);
}
no_of_guesses++;
} while(guess!=number);
return 0;
}
Output
The output screenshot for our number guessing game is:
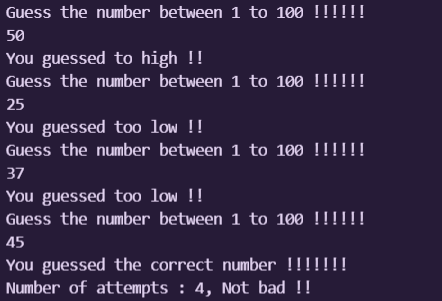
You may also like
Contacts Manager – Mini Project in C with source code
C++ Mini Project Bus Reservation System
Quiz Mini Project in Python using questions in JSON format
Card Game Mini Project in Python
Mini project Department Store System using C
Mini project Snake game using C
C Mini Project Ideas with a Sample Calculator Project
Computer Graphics (cg) mini project illumination model free download
Mini project Digital Volt Meter (DVM) using PIC16F877A with C code
Mini project Database Management System (DBMS) using wxWidget in C++
Mini project “3D Bounce” in OpenGL Utility Toolkit (GLUT) using C/C++ – Free Download
Mini project Banking Record System in C++
Mini project “Quiz (Who will be the millionaire)” Using C/C++ – Free download
Mini project “Library management system” using C – Free Code