Election Management System Mini Project using C with Source Code
Contents
Introduction
The C programming language is a procedural programming language. Between 1969 and 1973, Dennis Ritchie worked on the project. It was created primarily as a system programming language for developing operating systems. Low-level memory access, a small collection of keywords, and a clean style are all qualities that make C language excellent for system programming, such as operating system or compiler development.
Why Learn C Programming Language?
Because it mixes the characteristics of high-level languages with the functionalism of assembly language, C is often referred to as a middle-level computer language. The manipulation of bits, bytes, and addresses in C gives the programmer more control over how the program will behave and more direct access to the underlying hardware mechanisms.
Working programmers influenced, influenced, and field-tested C. As a result, C provides the programmer with exactly what he or she want. C++ is a more advanced version of the C programming language. C++ incorporates all of the features of C, as well as support for object-oriented programming (OOP). Furthermore, C++ includes numerous enhancements and features that make it a “better C,” regardless of whether or not it is used for object-oriented programming.
Election System
The best way to learn is to do. Hence, what better way to learn C programming language (if you know the basics) than to code your first mini project. For this blog we are going to build a election management system mini project using c programming language.
In order to make our election system we use the following concepts of C programming language:
- Multiple headers.
#include <stdio.h>
#include <stdlib.h>
- Use of loops
// use of loop in our code:
if(vote_Count1 > vote_Count2 && vote_Count1 > vote_Count3 && vote_Count1 > vote_Count4){
printf("The Leading Candiate is %s", party_no_1);
}else if(vote_Count2 > vote_Count1 && vote_Count2 > vote_Count3 && vote_Count2 > vote_Count4){
printf("The Leading Candiate is %s", party_no_2);
}else if(vote_Count3 > vote_Count2 && vote_Count3 > vote_Count1 && vote_Count3 > vote_Count4){
printf("The Leading Candiate is %s", party_no_3);
}else if(vote_Count4 > vote_Count2 && vote_Count4> vote_Count3 && vote_Count4 > vote_Count1){
printf("The Leading Candiate is %s", party_no_4);
}else {
printf("+++++++++++++ Warning!!!!!!!!! No Win-Win Situation ++++++++++++++");
}
- use of functions.
// use of functions in our code
// user defined casteVote function:
void castVote(){
int choice;
printf("\n \n <#> <#> <#> <#> Please chooose your Candidate <#> <#> <#> <#> \n \n");
printf("\n 1. %s", party_no_1); ......
}
// user defined votesCount function
void votesCount(){
printf("\n \n #_#_#_#_# Voting Statics #_#_#_#_#");
...........
}
// user defined getLeadingCandiate function
void getLeadingCandiate(){
printf("\n \n #_#_#_#_# Leading Candiate #_#_#_#_#");
................
}
- use of switch cases.
// use of switch cases in our code
switch (choice){
case 1:
vote_Count1++;
break;
case 2:
vote_Count2++;
break;
case 3:
vote_Count3++;
break;
case 4:
vote_Count4++;
break;
case 5:
invalid_votes++;
break;
default:
printf(" \n Error: Wrong Chocie !! Please retry");
- use of define preprocessor.
// define macroname charseq
// total no of the parties
#define total_no_of_parties
#define party_no_1 "A"
#define party_no_2 "B"
#define party_no_3 "C"
#define party_no_4 "D"
#include <stdio.h>
#include <stdlib.h>
// define macroname charseq
// total no of the parties
#define total_no_of_parties
#define party_no_1 "A"
#define party_no_2 "B"
#define party_no_3 "C"
#define party_no_4 "D"
int vote_Count1 = 0,
vote_Count2 = 0,
vote_Count3 = 0,
vote_Count4 = 0,
invalid_votes = 0;
void castVote(){
int choice;
printf("\n \n <#> <#> <#> <#> Please chooose your Candidate <#> <#> <#> <#> \n \n");
printf("\n 1. %s", party_no_1);
printf("\n 2. %s", party_no_2);
printf("\n 3. %s", party_no_3);
printf("\n 4. %s", party_no_4);
printf("\n 5. %s", "None of the above");
printf("\n \n Input your choice ( 1 - 4 ) : ");
scanf("%d", &choice);
switch (choice){
case 1:
vote_Count1++;
break;
case 2:
vote_Count2++;
break;
case 3:
vote_Count3++;
break;
case 4:
vote_Count4++;
break;
case 5:
invalid_votes++;
break;
default:
printf(" \n Error: Wrong Chocie !! Please retry");
// built in function for holding the screen.
getchar();
}
printf(" \n Thanks for your vote !!");
}
void votesCount(){
printf("\n \n #_#_#_#_# Voting Statics #_#_#_#_#");
printf("\n %s - %d", party_no_1, vote_Count1);
printf("\n %s - %d", party_no_2, vote_Count2);
printf("\n %s - %d", party_no_3, vote_Count3);
printf("\n %s - %d", party_no_4, vote_Count4);
printf("\n %s - %d", "Spoiled Votes", invalid_votes);
}
void getLeadingCandiate(){
printf("\n \n #_#_#_#_# Leading Candiate #_#_#_#_#");
if(vote_Count1 > vote_Count2 && vote_Count1 > vote_Count3 && vote_Count1 > vote_Count4){
printf("The Leading Candiate is %s", party_no_1);
}else if(vote_Count2 > vote_Count1 && vote_Count2 > vote_Count3 && vote_Count2 > vote_Count4){
printf("The Leading Candiate is %s", party_no_2);
}else if(vote_Count3 > vote_Count2 && vote_Count3 > vote_Count1 && vote_Count3 > vote_Count4){
printf("The Leading Candiate is %s", party_no_3);
}else if(vote_Count4 > vote_Count2 && vote_Count4> vote_Count3 && vote_Count4 > vote_Count1){
printf("The Leading Candiate is %s", party_no_4);
}else {
printf("+++++++++++++ Warning!!!!!!!!! No Win-Win Situation ++++++++++++++");
}
}
int main(){
int choice;
do{
printf("\n \n #_#_#_#_#_# WELCOME TO ELECTION / VOTING 2022 A.D #_#_#_#_#_#");
printf("\n \n 1. Cast the Vote.");
printf("\n \n 2. Find the Vote Count.");
printf("\n \n 3. Find the Leading Candidate.");
printf("\n \n 0. Exit.");
printf("\n \n Please Enter Your Choice : ");
scanf("%d", &choice);
switch(choice){
case 1:
castVote();
break;
case 2:
votesCount();
break;
case 3:
getLeadingCandiate();
break;
default:
printf("\n Error: Invalid Choice !!!");
}
} while (choice!=0);
getchar();
}
Pros and Cons of our Election System
Pros
- Reusable code that can be adapted into other systems.
- Reprogrammable according to the particular programmers taste.
- Better and more efficient in comparison with traditional voting system.
Cons
- No GUI (Graphical User Interface).
- Can be more secure.
Output
The following are the output for our election management system:
- Our Election Management systems’ front page / main page / menu.
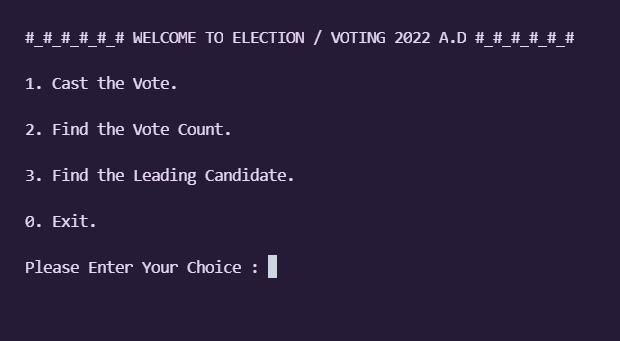
- Choice 1, “Cast the Vote.”
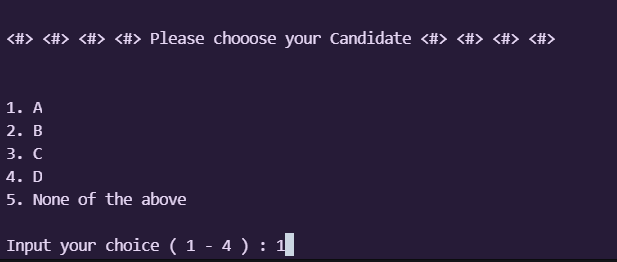
- Choice 2, “Find the Vote Count.”
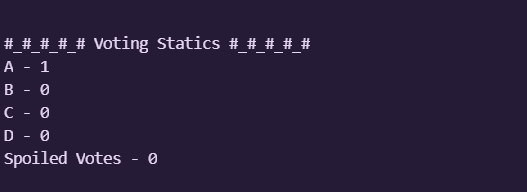
- Choice 3, “Find the Leading Candidate.”

- Choice 0, “Exit.”. This choice pretty much returns an error as Invalid Choice and exits.
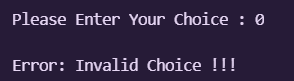
You may also like
Contacts Manager – Mini Project in C with source code
C++ Mini Project Bus Reservation System
Quiz Mini Project in Python using questions in JSON format
Card Game Mini Project in Python
Mini project Department Store System using C
Mini project Snake game using C
C Mini Project Ideas with a Sample Calculator Project
Computer Graphics (cg) mini project illumination model free download
Mini project Digital Volt Meter (DVM) using PIC16F877A with C code
Mini project Database Management System (DBMS) using wxWidget in C++
Mini project “3D Bounce” in OpenGL Utility Toolkit (GLUT) using C/C++ – Free Download
Mini project Banking Record System in C++
Mini project “Quiz (Who will be the millionaire)” Using C/C++ – Free download
Mini project “Library management system” using C – Free Code