Drawing Square and Rectangle Patterns in C Program
Contents
Introduction
The C programming language is a procedural programming language. Between 1969 and 1973, Dennis Ritchie worked on the project. It was created primarily as a system programming language for developing operating systems.
In this tutorial we are going to learn to draw or print different variations of Square and Rectangle patterns using C programming language.
In order to understand the code given below you’ve got to have prior knowledge and or understanding of the following topics:
If you have a sound knowledge and understanding of what the functions of the concepts are then there will be no difficulty in understanding the given codes for square and rectangle patterns in c programming language. With all that said lets get coding!
Square and Rectangle made of *
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
// Square and Rectangle made of *
int main()
{
int n;
printf("Enter the number of rows: ");
scanf("%d",&n);
for(int r=1; r<=n; r++)
{
for(int c=1; c<=n; c++)
{
printf("* ");
}
printf("\n");
}
return 0;
}
Output for Square and Rectangle made of *
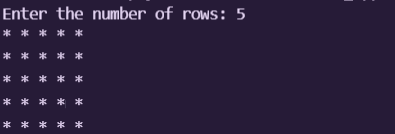
Square and Rectangle made of Numbers
Method 1 of making Square and Rectangle made of Numbers
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
// method 1 of drawing square with numbers
// in this case the square is made up of numbers ...
// ... that are in ascending order in the row
int main()
{
int n;
printf("Enter the number of rows: ");
scanf("%d",&n);
for(int r=1; r<=n; r++)
{
for(int c=1; c<=n; c++)
{
printf("%3d",c);
}
printf("\n");
}
return 0;
}
Output for Method 1 of making Square and Rectangle made of Numbers
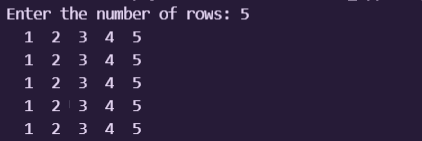
Method 2 of making Square and Rectangle made of Numbers
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
// method 2 of drawing square with numbers
// in this case the square is made up of numbers ...
// ... that are in ascending order in the column
int main()
{
int n;
printf("Enter the number of rows: ");
scanf("%d",&n);
for(int r=1; r<=n; r++)
{
for(int c=1; c<=n; c++)
{
printf("%3d",r);
}
printf("\n");
}
return 0;
}
Output for Method 2 of making Square and Rectangle made of Numbers
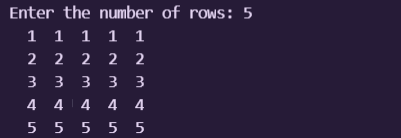
Square and Rectangle made of Alphabets
Method 1 of making Square and Rectangle made of Alphabets
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
// method 1 of drawing square with alphabets in lowercase
// In this case the square is made up of alphabets ...
// ... that are in ascending order in the row
int main()
{
int n;
printf("Enter the number of rows: ");
scanf("%d",&n);
for(int r=1; r<=n; r++)
{
for(int c=1; c<=n; c++)
{
printf("%3c",c+96);
}
printf("\n");
}
return 0;
}
Output for Method 1 of making Square and Rectangle made of Alphabets
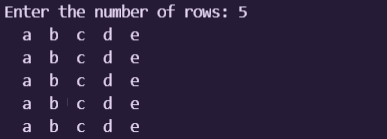
Method 2 of making Square and Rectangle made of Alphabets
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
// method 2 of drawing square with alphabets in uppercase
// In this case the square is made up of alphabets ...
// ... that are in ascending order in the row
int main()
{
int n;
printf("Enter the number of rows: ");
scanf("%d", &n);
for(int r=1; r<=n; r++)
{
for(int c=1; c<=n; c++)
{
printf("%3c",c+64);
}
printf("\n");
}
return 0;
}
Output for Method 2 of making Square and Rectangle made of Alphabets
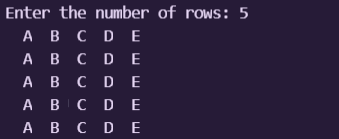
Method 3 of making Square and Rectangle made of Alphabets
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
// method 3 of drawing square with alphabets in lowercase
// In this case the square is made up of alphabets ...
// ... that are in ascending order in the column
int main()
{
int n;
printf("Enter number of rows: ");
scanf("%d",&n);
for(int r=1; r<=n; r++)
{
for(int c=1; c<=n; c++)
{
printf("%3c",r+96);
}
printf("\n");
}
return 0;
}
Output for Method 3 of making Square and Rectangle made of Alphabets
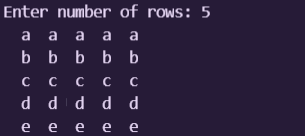
Method 4 of making Square and Rectangle made of Alphabets
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
// method 4 of drawing square with alphabets in uppercase
// In this case the square is made up of alphabets ...
// ... that are in ascending order in the column
int main()
{
int n;
printf("Enter number of rows: ");
scanf("%d",&n);
for(int r=1; r<=n; r++)
{
for(int c=1; c<=n; c++)
{
printf("%3c",r+64);
}
printf("\n");
}
return 0;
}
Output for Method 4 of making Square and Rectangle made of Alphabets
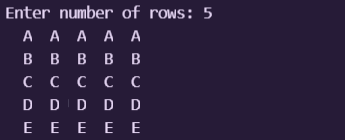
Method 5 of making Square and Rectangle made of Alphabets
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
// method 5 of drawing square with alphabets in lowercase and uppercase alternatingly
// In this case the square is made up of alphabets ...
// ... that are in ascending order in the column
int main()
{
int n;
printf("Enter the number of rows: ");
scanf("%d", &n);
for(int r=1; r<=n; r++)
{
for(int c=1; c<=n; c++)
{
if(r%2==0) printf("%3c", r+64);
else printf("%3c", r+96);
}
printf("\n");
}
return 0;
}
Output for Method 5 of making Square and Rectangle made of Alphabets
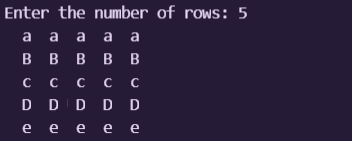
Square Pattern drawn with a border made of *
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
// Square Pattern drawn with a border made of *
// Square Pattern is in a continuous sequence and doesn't start from A every row
// if number of rows is 3 the first row is A B C and the second row is D E F
// see the output below
int main()
{
int n,r,c;
char ch = 'A';
printf("Enter number of rows: ");
scanf("%d",&n);
// using nested loops
// the outer loop
for(r=1; r<=n; r++)
{
// the inner loop
for(c=1; c<=n; c++)
{
if(c==1||r==1||c==n||r==n) printf("* ");
else printf("%c ",ch++);
if(ch > 'Z') ch='A';
} // the end of inner loop
printf("\n");
} // the eend of outer loop
return 0;
}
Output for Square Pattern drawn with a border made of *
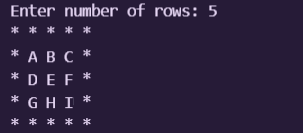