Calculator Mini Project using Flutter with Source Code
In this tutorial we are going to make a simple calculator flutter app In the modern day caring a physical calculator is seem more as a hassle. It is for this reason that they have been mostly replaced by software calculators. If we think about it all consumer devices from our desktop to mobiles have a inbuilt calculator app. As the saying goes, “the best way to learn is to do”, let’s sharpen our flutter skills by making this simple flutter app.
Simple Flutter Calculator App
The goal of a calculator is to perform accurate calculations quickly. It is obvious that, to the extent possible, a calculator should relieve the user of the need to perform mental calculations and rely on paper.
With that said, Let’s get coding!
- Create a flutter app using
flutter create flutter_calculator
. This command creates a flutter application named flutter_calculator.
Dependencies
- Paste the following code in your
pubspec.yaml
file:
name: ornekler
description: A new Flutter project.
# The following line prevents the package from being accidentally published to
# pub.dev using `flutter pub publish`. This is preferred for private packages.
publish_to: 'none' # Remove this line if you wish to publish to pub.dev
# The following defines the version and build number for your application.
# A version number is three numbers separated by dots, like 1.2.43
# followed by an optional build number separated by a +.
# Both the version and the builder number may be overridden in flutter
# build by specifying --build-name and --build-number, respectively.
# In Android, build-name is used as versionName while build-number used as versionCode.
# Read more about Android versioning at https://developer.android.com/studio/publish/versioning
# In iOS, build-name is used as CFBundleShortVersionString while build-number used as CFBundleVersion.
# Read more about iOS versioning at
# https://developer.apple.com/library/archive/documentation/General/Reference/InfoPlistKeyReference/Articles/CoreFoundationKeys.html
version: 1.0.0+1
environment:
sdk: ">=2.15.0 <3.0.0"
# Dependencies specify other packages that your package needs in order to work.
# To automatically upgrade your package dependencies to the latest versions
# consider running `flutter pub upgrade --major-versions`. Alternatively,
# dependencies can be manually updated by changing the version numbers below to
# the latest version available on pub.dev. To see which dependencies have newer
# versions available, run `flutter pub outdated`.
dependencies:
flutter:
sdk: flutter
# The following adds the Cupertino Icons font to your application.
# Use with the CupertinoIcons class for iOS style icons.
cupertino_icons: ^1.0.2
dev_dependencies:
flutter_test:
sdk: flutter
# The "flutter_lints" package below contains a set of recommended lints to
# encourage good coding practices. The lint set provided by the package is
# activated in the `analysis_options.yaml` file located at the root of your
# package. See that file for information about deactivating specific lint
# rules and activating additional ones.
flutter_lints: ^1.0.0
# For information on the generic Dart part of this file, see the
# following page: https://dart.dev/tools/pub/pubspec
# The following section is specific to Flutter.
flutter:
# The following line ensures that the Material Icons font is
# included with your application, so that you can use the icons in
# the material Icons class.
uses-material-design: true
# To add assets to your application, add an assets section, like this:
# assets:
# - images/a_dot_burr.jpeg
# - images/a_dot_ham.jpeg
# An image asset can refer to one or more resolution-specific "variants", see
# https://flutter.dev/assets-and-images/#resolution-aware.
# For details regarding adding assets from package dependencies, see
# https://flutter.dev/assets-and-images/#from-packages
# To add custom fonts to your application, add a fonts section here,
# in this "flutter" section. Each entry in this list should have a
# "family" key with the font family name, and a "fonts" key with a
# list giving the asset and other descriptors for the font. For
# example:
# fonts:
# - family: Schyler
# fonts:
# - asset: fonts/Schyler-Regular.ttf
# - asset: fonts/Schyler-Italic.ttf
# style: italic
# - family: Trajan Pro
# fonts:
# - asset: fonts/TrajanPro.ttf
# - asset: fonts/TrajanPro_Bold.ttf
# weight: 700
#
# For details regarding fonts from package dependencies,
# see https://flutter.dev/custom-fonts/#from-packagess
Source Code
- Go inside the flutter_calculator folder.
- Inside the lib folder paste the following code in
main.dart
lib/main.dart
import 'package:flutter/material.dart';
void main() => runApp(new MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return new MaterialApp(
title: 'Flutter Demo',
theme: new ThemeData(
primarySwatch: Colors.blue,
),
home: new MyHomePage(title: 'Calculator'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => new _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
String output = "0";
String _output = "0";
double num1 = 0.0;
double num2 = 0.0;
String operand = "";
buttonPressed(String buttonText){
if(buttonText == "CLEAR"){
_output = "0";
num1 = 0.0;
num2 = 0.0;
operand = "";
} else if (buttonText == "+" || buttonText == "-" || buttonText == "/" || buttonText == "X"){
num1 = double.parse(output);
operand = buttonText;
_output = "0";
} else if(buttonText == "."){
if(_output.contains(".")){
print("Already conatains a decimals");
return;
} else {
_output = _output + buttonText;
}
} else if (buttonText == "="){
num2 = double.parse(output);
if(operand == "+"){
_output = (num1 + num2).toString();
}
if(operand == "-"){
_output = (num1 - num2).toString();
}
if(operand == "X"){
_output = (num1 * num2).toString();
}
if(operand == "/"){
_output = (num1 / num2).toString();
}
num1 = 0.0;
num2 = 0.0;
operand = "";
} else {
_output = _output + buttonText;
}
print(_output);
setState(() {
output = double.parse(_output).toStringAsFixed(2);
});
}
Widget buildButton(String buttonText) {
return new Expanded(
child: new OutlineButton(
padding: new EdgeInsets.all(24.0),
child: new Text(buttonText,
style: TextStyle(
fontSize: 20.0,
fontWeight: FontWeight.bold
),
),
onPressed: () =>
buttonPressed(buttonText)
,
),
);
}
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: new AppBar(
title: new Text(widget.title),
),
body: new Container(
child: new Column(
children: <Widget>[
new Container(
alignment: Alignment.centerRight,
padding: new EdgeInsets.symmetric(
vertical: 24.0,
horizontal: 12.0
),
child: new Text(output, style: new TextStyle(
fontSize: 48.0,
fontWeight: FontWeight.bold,
))),
new Expanded(
child: new Divider(),
),
new Column(children: [
new Row(children: [
buildButton("7"),
buildButton("8"),
buildButton("9"),
buildButton("/")
]),
new Row(children: [
buildButton("4"),
buildButton("5"),
buildButton("6"),
buildButton("X")
]),
new Row(children: [
buildButton("1"),
buildButton("2"),
buildButton("3"),
buildButton("-")
]),
new Row(children: [
buildButton("."),
buildButton("0"),
buildButton("00"),
buildButton("+")
]),
new Row(children: [
buildButton("CLEAR"),
buildButton("="),
])
])
],
)));
}
}
Output
The output for the Calculator mini project in flutter is given below:
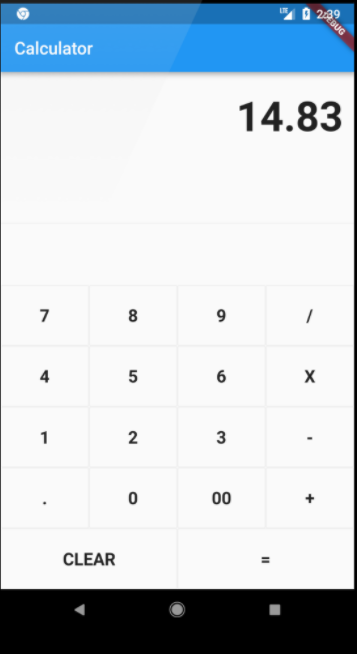