Drawing Diamond Patterns in C Program
Introduction
The C programming language is a procedural programming language. Between 1969 and 1973, Dennis Ritchie worked on the project. It was created primarily as a system programming language for developing operating systems.
In this tutorial we are going to learn to draw or print different variation of diamond pattern using C programming language.
In order to understand the code given below you’ve got to have prior knowledge and or understanding of the following topics:
If you have a sound knowledge and understanding of what the functions of the concepts are then there will be no difficulty in understanding the given codes for different variation of diamond pattern in c programming language. With all that said lets get coding!
Diamond made of *
The following how we will make a program that prompts the user for the upper-half diamond’s row size before printing the diamond pattern of stars. For example, if the user selects 5 as the row size, a diamond of stars with a dimension of 5*2-1 or 9 rows is displayed. This same concept applies for all the other diamond variations presented here in this tutorial.
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
int main()
{
int i, j, row, space;
printf("Enter Number of Rows: ");
scanf("%d", &row);
space = row-1;
for(i=1; i<=row; i++)
{
for(j=1; j<=space; j++)
printf(" ");
space--;
for(j=1; j<=(2*i-1); j++)
printf("*");
printf("\n");
}
space = 1;
for(i=1; i<=(row-1); i++)
{
for(j=1; j<=space; j++)
printf(" ");
space++;
for(j=1; j<=(2*(row-i)-1); j++)
printf("*");
printf("\n");
}
getch();
return 0;
}
Output for Diamond made of *
To print a diamond design that spreads to the row-1 line, input the number of rows, say 7. That is, with row number 7, it will print a 6-line diamond pattern, as demonstrated in the output below:
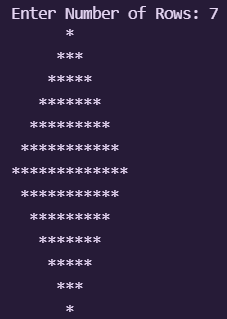
Diamond made of Numbers
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
int main()
{
int i, j, row, space, num=1;
printf("Enter Number of Rows: ");
scanf("%d", &row);
space = row-1;
for(i=1; i<=row; i++)
{
for(j=1; j<=(space); j++)
printf(" ");
space--;
for(j=1; j<=(2*i-1); j++)
{
printf("%d", num);
num++;
}
printf("\n");
num=1;
}
space = 1;
for(i=1; i<=(row-1); i++)
{
for(j=1; j<=(space); j++)
printf(" ");
space++;
for(j=1; j<=(2*(row-i)-1); j++)
{
printf("%d", num);
num++;
}
printf("\n");
num=1;
}
getch();
return 0;
}
Output for Diamond made of Numbers
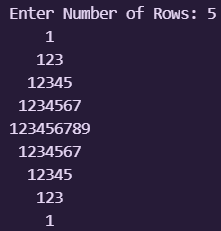
Diamond made of Alphabets
Method 1 for Diamond made of Alphabets
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
// c program for printing a diamond pattern ....
// ... made with lowercase alphabets
int main()
{
int i, j, row, space;
char ch='A';
printf("Enter Number of Rows: ");
scanf("%d", &row);
space = row-1;
for(i=1; i<=row; i++)
{
for(j=1; j<=(space); j++)
printf(" ");
space--;
for(j=1; j<=(2*i-1); j++)
{
printf("%c", ch);
ch++;
}
ch='A';
printf("\n");
}
space = 1;
for(i=1; i<=(row-1); i++)
{
for(j=1; j<=(space); j++)
printf(" ");
space++;
for(j=1; j<=(2*(row-i)-1); j++)
{
printf("%c", ch);
ch++;
}
ch='A';
printf("\n");
}
getch();
return 0;
}
Output for Diamond made of Alphabets
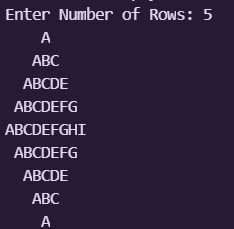
Method 2 Diamond made of Alphabets
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
// c program for printing a diamond pattern ....
// ... made with lowercase alphabets
int main()
{
int i, j, row, space;
char ch='a';
printf("Enter Number of Rows: ");
scanf("%d", &row);
space = row-1;
for(i=1; i<=row; i++)
{
for(j=1; j<=(space); j++)
printf(" ");
space--;
for(j=1; j<=(2*i-1); j++)
{
printf("%c", ch);
ch++;
}
ch='a';
printf("\n");
}
space = 1;
for(i=1; i<=(row-1); i++)
{
for(j=1; j<=(space); j++)
printf(" ");
space++;
for(j=1; j<=(2*(row-i)-1); j++)
{
printf("%c", ch);
ch++;
}
ch='a';
printf("\n");
}
getch();
return 0;
}
Output for Diamond made of Alphabets
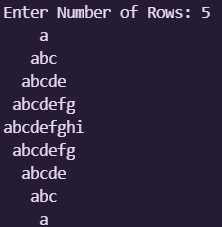