Drawing Pyramid Patterns in C Program
Contents
- 1 Introduction
- 2 Half Pyramid made of *
- 3 Half Pyramid made of numbers
- 4 Half Pyramid made of Alphabets
- 5 Inverted Half Pyramid of *
- 6 Inverted Half Pyramid of numbers
- 7 Inverted Half Pyramid made of Alphabets
- 8 Full Pyramid of *
- 9 Full Pyramid made of numbers
- 10 Full Pyramid made of Alphabets
- 11 Inverted Full Pyramid made of *
- 12 Inverted Full Pyramid made of numbers
- 13 Inverted Full Pyramid made of Alphabets
Introduction
The C programming language is a procedural programming language. Between 1969 and 1973, Dennis Ritchie worked on the project. It was created primarily as a system programming language for developing operating systems.
In this tutorial we are going to learn to draw or print pyramids and patterns using C programming language.
In order to understand the code given below you’ve got to have prior knowledge and or understanding of the following topics:
If you have a sound knowledge and understanding of what the functions of the concepts are then there will be no difficulty in understanding the given codes for patterns and pyramids in c programming language. With all that said lets get coding!
Half Pyramid made of *
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
int main() {
int i, j, rows;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = 1; i <= rows; ++i) {
for (j = 1; j <= i; ++j) {
printf("* ");
}
printf("\n");
}
return 0;
}
Output for Half Pyramid made of *
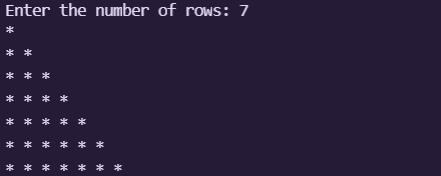
Half Pyramid made of numbers
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
int main() {
int i, j, rows;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = 1; i <= rows; ++i) {
for (j = 1; j <= i; ++j) {
printf("%d ", j);
}
printf("\n");
}
return 0;
}
Output for Half Pyramid made of numbers
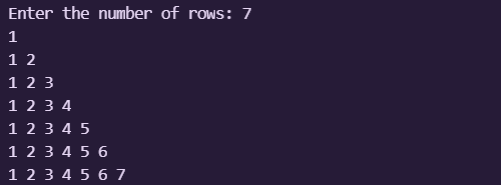
Half Pyramid made of Alphabets
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
int main() {
int i, j;
char input, alphabet = 'a'; // for capital characters use alphabet = 'A'
printf("Enter an lowercase character you want to print in the last row: ");
scanf("%c", &input);
for (i = 1; i <= (input - 'a' + 1); ++i) {
for (j = 1; j <= i; ++j) {
printf("%c ", alphabet);
}
++alphabet;
printf("\n");
}
return 0;
}
Output for Half Pyramid made of Alphabets
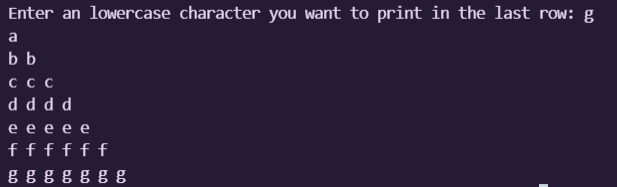
Inverted Half Pyramid of *
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
int main() {
int i, j, rows;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = rows; i >= 1; --i) {
for (j = 1; j <= i; ++j) {
printf("* ");
}
printf("\n");
}
return 0;
}
Output for Inverted Half Pyramid of *
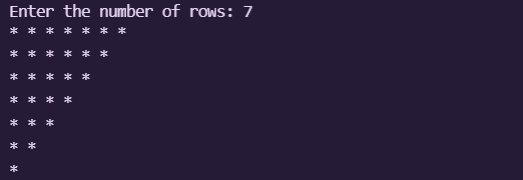
Inverted Half Pyramid of numbers
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
int main() {
int i, j, rows;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = rows; i >= 1; --i) {
for (j = 1; j <= i; ++j) {
printf("%d ", j);
}
printf("\n");
}
return 0;
}
Output for Inverted Half Pyramid of numbers
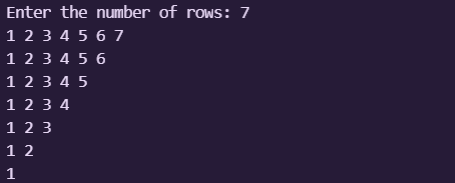
Inverted Half Pyramid made of Alphabets
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
int main()
{
int i,j,a;
char c='a';
printf("Enter the number of rows: ");
scanf("%d", &a);
for(i=a;i>0;i--)
{
for(j=i;j>=1;j--)
{
printf("%c",c);
}
c++;
printf("\n");
}
return 0;
}
Output for Inverted Half Pyramid made of Alphabets
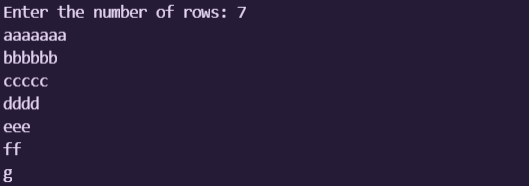
Full Pyramid of *
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
int main() {
int i, space, rows, k = 0;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = 1; i <= rows; ++i, k = 0) {
for (space = 1; space <= rows - i; ++space) {
printf(" ");
}
while (k != 2 * i - 1) {
printf("* ");
++k;
}
printf("\n");
}
return 0;
}
Output for Full Pyramid of *
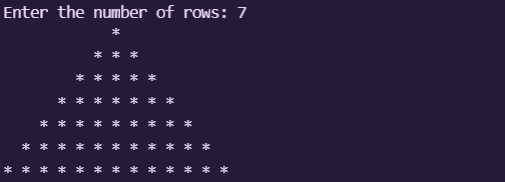
Full Pyramid made of numbers
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
int main() {
int i, space, rows, k = 0, count = 0, count1 = 0;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = 1; i <= rows; ++i) {
for (space = 1; space <= rows - i; ++space) {
printf(" ");
++count;
}
while (k != 2 * i - 1) {
if (count <= rows - 1) {
printf("%d ", i + k);
++count;
} else {
++count1;
printf("%d ", (i + k - 2 * count1));
}
++k;
}
count1 = count = k = 0;
printf("\n");
}
return 0;
}
Output for Full Pyramid made of numbers
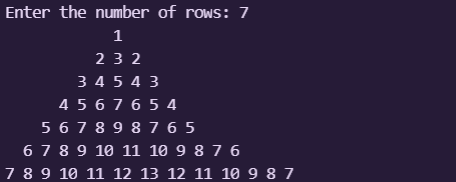
Full Pyramid made of Alphabets
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
void main()
{
int i, j;
char alph = 'a';
int n,blk;
int ctr = 1;
printf("Enter the number of Letters (less than 26) in the Pyramid : ");
scanf("%d", &n);
for (i = 1; i <= n; i++)
{
for(blk=1;blk<=n-i;blk++)
printf(" ");
for (j = 0; j <= (ctr / 2); j++) {
printf("%c ", alph++);
}
alph = alph - 2;
for (j = 0; j < (ctr / 2); j++) {
printf("%c ", alph--);
}
ctr = ctr + 2;
alph = 'a';
printf("\n");
}
}
Output for Full Pyramid made of Alphabets
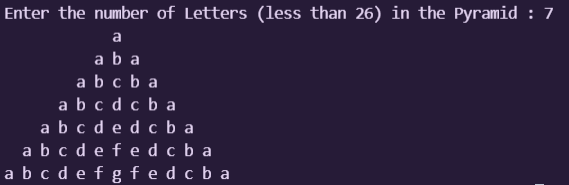
Inverted Full Pyramid made of *
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
int main() {
int rows, i, j, space;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = rows; i >= 1; --i) {
for (space = 0; space < rows - i; ++space)
printf(" ");
for (j = i; j <= 2 * i - 1; ++j)
printf("* ");
for (j = 0; j < i - 1; ++j)
printf("* ");
printf("\n");
}
return 0;
}
Output for Inverted Full Pyramid made of *
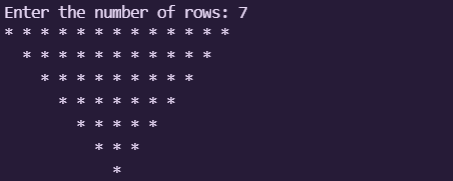
Inverted Full Pyramid made of numbers
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
int main()
{
int i,j,rows,space=0;
printf("Enter the number of rows: ");
scanf("%d",&rows);
for(i=rows; i>=1; i--){
for(j=1; j<=space; j++)
printf(" ");
for(j=1; j<=i; j++)
printf("%d",j);
for(j=i-1; j>=1; j--)
printf("%d",j);
printf("\n");
space++;
}
getch();
return 0;
}
Output for Inverted Full Pyramid made of numbers
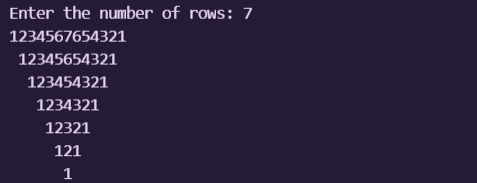
Inverted Full Pyramid made of Alphabets
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
int main()
{
printf("Enter the number of row (upto 26): ");
int row;
scanf("%d",&row);
int np=row*2-1,in,out,p;
for(out=row-1;out>=0;out--)
{
for(in=row-1;in>out;in--)
printf(" ");
for(p=0;p<np;p++)
printf("%c",(out+65));
np-=2;
printf("\n");
}
}
Output for Inverted Full Pyramid made of Alphabets
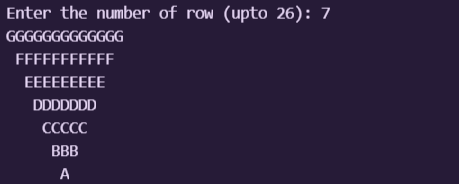