Drawing Pascal’s Triangle and Floyd’s Triangle using C Program
Introduction
The C programming language is a procedural programming language. Between 1969 and 1973, Dennis Ritchie worked on the project. It was created primarily as a system programming language for developing operating systems.
In this tutorial we are going to learn to draw or print Pascal’s Triangle and Floyd’s Triangle using C programming language.
In order to understand the code given below you’ve got to have prior knowledge and or understanding of the following topics:
If you have a sound knowledge and understanding of what the functions of the concepts are then there will be no difficulty in understanding the given codes for Pascal’s Triangle and Floyd’s Triangle in c programming language. With all that said lets get coding!
Pascal’s Triangle
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
int main() {
int rows, coef = 1, space, i, j;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = 0; i < rows; i++) {
for (space = 1; space <= rows - i; space++)
printf(" ");
for (j = 0; j <= i; j++) {
if (j == 0 || i == 0)
coef = 1;
else
coef = coef * (i - j + 1) / j;
printf("%4d", coef);
}
printf("\n");
}
return 0;
}
Output for Pascal’s Triangle
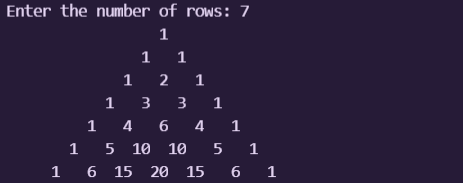
Floyd’s Triangle
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
int main() {
int rows, i, j, number = 1;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = 1; i <= rows; i++) {
for (j = 1; j <= i; ++j) {
printf("%d ", number);
++number;
}
printf("\n");
}
return 0;
}
Output for Floyd’s Triangle
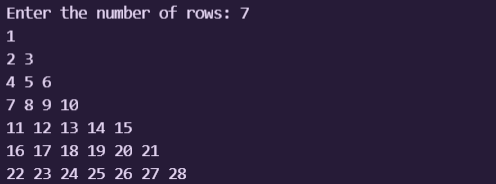