Number Guessing Mini Project using Python3 with Source Code
Contents
Introduction
Python is a high-level, general-purpose programming language that is interpreted. The use of considerable indentation in its design philosophy emphasizes code readability. Its language elements and object-oriented approach are aimed at assisting programmers in writing clear, logical code for both small and large-scale projects.
Why Should I Learn Python 3 ?
Because of its adaptability, flexibility, and object-oriented characteristics, Python is one of the most popular programming languages among developers, data scientists, software engineers, and even hackers. Python’s rich libraries, frameworks, massive collections of modules, and file extensions are responsible for many of the web and mobile apps we use today. Python is also useful for developing micro-projects to large-scale enterprise online services, as well as supporting other programming languages.
Despite the fact that it is a high-level language capable of doing complex tasks, Python is simple to learn and has a simple syntax. As a result, it is suitable for both novice and professional programmers. There are numerous ways to learn Python, including self-study, enrolling in a coding bootcamp, or hiring a tutor.
Number Guessing Game
The best way to learn is to do. Hence, what better way to learn python programming language (if you know the basics) than to code your first mini project. For this blog we are going to build number guessing game mini project using python programming language. Keep in mind this is a mini project for absolute beginners and intermediate programmers as well.
Explanation
The concept for our guessing game can be understood using the below example
An example of how our game works:
Player A: I am thinking of a number from 1 to 100, can you guess my number within 4 turns ?
Player B: Sure, is your number 50 ?
Player A: Nope too high !!.
Player B: Okay, is your number 25 ?
Player A: Nope, guess a higher number.
Player B: Okay is your number 37 ?
Player A: Nope, guess a higher number.
Player B: Okay, is your number 45 ?
Player A: Yes, congratulations you guessed my number in 4 turns you win!
Our Approach
- The user specifies the range’s lower and upper bounds.
- The compiler creates a random integer in the range and saves it in a variable for future use.
- A while loop will be set up to allow for repeated guessing.
- If the user guesses a number that is higher than a randomly chosen number, the user is given the warning “You guessed too high !!”
- Else If the user guesses a number that is less than the randomly chosen number, the user receives the message “You guessed too low !!”
- And if the user guessed in a minimum number of guesses, the user gets a congratulatory “You guessed the correct number !!!!!!!” output along with the number of guesses.
- If the user does not guess the integer in the required amount of guesses, he or she will receive the message “Better Luck Next Time!”
Source Code
import random
import math
# Taking Inputs
lower = int(input("Enter Lower bound:- "))
# Taking Inputs
upper = int(input("Enter Upper bound:- "))
# generating random number between
# the lower and upper
x = random.randint(lower, upper)
print("\n\tYou've only ",
round(math.log(upper - lower + 1, 2)),
" chances to guess the integer!\n")
# Initializing the number of guesses.
count = 0
# for calculation of minimum number of
# guesses depends upon range
while count < math.log(upper - lower + 1, 2):
count += 1
# taking guessing number as input
guess = int(input("Guess a number:- "))
# Condition testing
if x == guess:
print("Congratulations you did it in ",
count, " try")
# Once guessed, loop will break
break
elif x > guess:
print("You guessed too small!")
elif x < guess:
print("You Guessed too high!")
# If Guessing is more than required guesses,
# shows this output.
if count >= math.log(upper - lower + 1, 2):
print("\nThe number is %d" % x)
print("\tBetter Luck Next time!")
Output
The following is the output for the given code above:
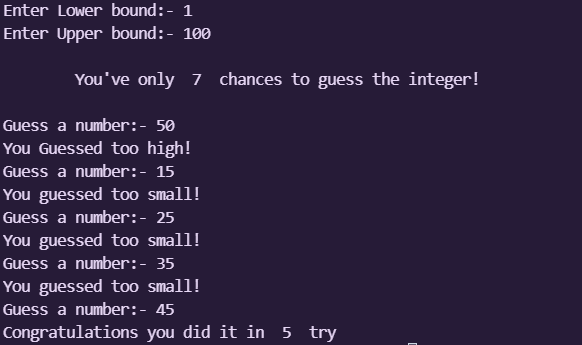
You may also like: Number Guessing Mini Project using C with Source Code.