Drawing Hollow Patterns in C Program
Introduction
The C programming language is a procedural programming language. Between 1969 and 1973, Dennis Ritchie worked on the project. It was created primarily as a system programming language for developing operating systems.
In this tutorial we are going to learn to draw or print different variation of hollow patterns using C programming language.
In order to understand the code given below you’ve got to have prior knowledge and or understanding of the following topics:
If you have a sound knowledge and understanding of what the functions of the concepts are then there will be no difficulty in understanding the given codes for different variation of hollow patterns in c programming language. With all that said lets get coding!
Printing Hollow Diamond of *
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
int main()
{
printf("Enter the number of rows : ");
int n, x, y, s = 1, k;
scanf("%d",&n);
for(x = 0; x <= n; x++)
{
for(y = n; y > x; y--)
{
printf(" ");
}
printf("*");
if (x > 0)
{
for(k = 1; k <= s; k++)
{
printf(" ");
}
s += 2;
printf("*");
}
printf("\n");
}
s -= 4;
for(x = 0; x <= n -1; x++)
{
for(y = 0; y <= x; y++)
{
printf(" ");
}
printf("*");
for(k = 1; k <= s; k++)
{
printf(" ");
}
s -= 2;
if(x != n -1)
{
printf ("*");
}
// code for ending line after each row
printf("\n");
}
return 0;
}
Output for Printing Hollow Diamond of *
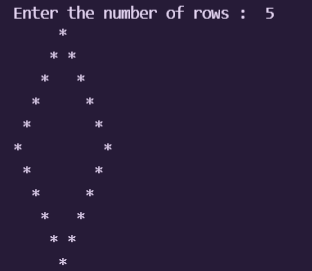
Printing Hollow Pyramid of *
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
int main()
{
int n, x, y, s;
printf("Enter number of rows : ");
scanf("%d",&n);
for(x = 1; x <= n; x++)
{
//for loop to put space in pyramid
for (s = x; s < n; s++)
printf(" "); //for loop to print star
for(y = 1; y <= (2 * n - 1); y++)
{
if(x == n || y == 1 || y == 2 * x - 1)
printf("*");
else
printf(" ");
}
//ending line after each row
printf("\n");
}
return 0;
}
Output for Hollow Pyramid of *
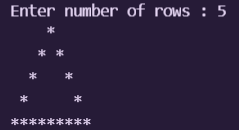