Fibonacci Series using Python3 with Source Code
Introduction
Python is a high-level, general-purpose programming language that is interpreted. The use of considerable indentation in its design philosophy emphasizes code readability. Its language elements and object-oriented approach are aimed at assisting programmers in writing clear, logical code for both small and large-scale projects.
Why Should I Learn Python 3 ?
Because of its adaptability, flexibility, and object-oriented characteristics, Python is one of the most popular programming languages among developers, data scientists, software engineers, and even hackers. Python’s rich libraries, frameworks, massive collections of modules, and file extensions are responsible for many of the web and mobile apps we use today. Python is also useful for developing micro-projects to large-scale enterprise online services, as well as supporting other programming languages.
Despite the fact that it is a high-level language capable of doing complex tasks, Python is simple to learn and has a simple syntax. As a result, it is suitable for both novice and professional programmers. There are numerous ways to learn Python, including self-study, enrolling in a coding bootcamp, or hiring a tutor.
Fibonacci Series
The Fibonacci numbers, typically abbreviated as Fₙ, are a mathematical series in which each number is the sum of the two preceding ones. The series usually begins with 0 and 1, while some authors skip the first two terms and begin with 1 and 1 or 1 and 2.
In C, the Fibonacci series defined the recurrence relation of numeric sequences.
It generates the next number by adding the second and third terms to the previous number instead of utilizing the first term. It can be done as the user requests till the number of terms is reached.
Except for the initial two numbers of the series (0, 1), every other third term is produced by adding the previous two numbers in Fibonacci sequence such as 0, 1, 1, 2, 3, 5, 8, 13, 21, and so on.
- Example 1: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34,
- Example 2: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597, 2584, 4181, 6765, 10946, 17711, 28657, 46368, 75025, 121393, 196418, 317811, …………
The best way to learn is to do. Hence, what better way to learn python3 language (if you know the basics) than to code a Fibonacci Series. In this tutorial we are going to code a Fibonacci Series using python3 language. Keep in mind this is a project for absolute beginners and intermediate programmers as well.
Source Code
# Program to display the Fibonacci sequence up to n-th term
# here n-th term is given or inputed by the user
nth_term = int(input("Enter the number of terms for our Fibonacci Series: "))
# first two terms
a, b = 0, 1
count = 0
# check if the number of terms is valid
if nth_term <= 0:
print("Please enter a positive integer")
# if there is only one term, return a
elif nth_term == 1:
print("Fibonacci sequence upto",nth_term,":")
print(a)
# generate fibonacci sequence
else:
print("Fibonacci sequence:")
while count < nth_term:
print(a)
nth = a + b
# update values
a = b
b = nth
count += 1
Output
The output screenshots for the above code is:
- Fibonacci Series using Python3 Screenshot 1:

- Fibonacci Series using Python3 Screenshot 2:
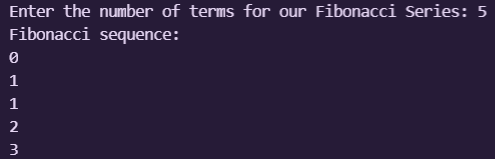
- Fibonacci Series using Python3 Screenshot 3:
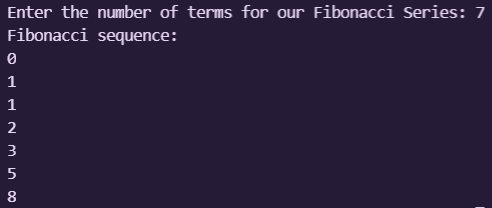